Overview
TheTracker is an application specifically built for NUS students. Allowing a student to keep track of his/her own occasions, modules and keep up to date with his/her friends.
Summary of contributions
-
Major enhancement: implemented the insertperson feature
-
What it does: Allows the user to build an association between a person and a module, or a person and an occasion.
-
Justification: This feature improves the product significantly as the user can now conveniently register his/her associated persons with modules and occasions. This makes TheTracker more of a holistic and useful product.
-
Highlights: This enhancement affects the existing delete and edit commands as now an edit to an entity must edit all the entities associated with it as well.
-
Credits: {All abstractions, logic was conceived of solely by me}
-
-
Minor enhancement: Added the new UI panels to display the contents of modules and occasions.
-
Code contributed: [Contributed Code]
-
Other contributions:
-
Project management:
-
Managed releases
v1.1
-v1.2
(2 releases) on GitHub and managed Pull Request to upstream CS2103 Main Repo for assessment by tutors.
-
-
Tools:
-
Integrated Travis CI into the repo.
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Inserting a Person: insertperson
Inserting a person into a module: insertperson
A command to allow the user to build an association between a module and a person within TheTracker
by inserting
a person
bi-directionally into a module
.
Format:
insertperson pi/PERSON_INDEX mi/MODULE_INDEX
Example:
-
insertperson pi/1 mi/1
Builds an association between the firstperson
andmodule
by insertingperson
1 bi-directionally intomodule
1.
Inserting a person into an occasion: insertperson
Builds an association between an occasion and a person within TheTracker
by inserting
a person
bi-directionally into an occasion
.
Format:
insertperson pi/PERSON_INDEX oi/OCCASION_INDEX
Example:
-
insertperson pi/1 oi/1
Builds an association between the firstperson
andoccasion
by insertingperson
1 bi-directionally intooccasion
1.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
To facilitate this the underlying structure of Person
, Module
and Occasion
have to
be linked in a manner that will facilitate injections of one entity bi-directionally into another.
The following class diagram depicts this structure:
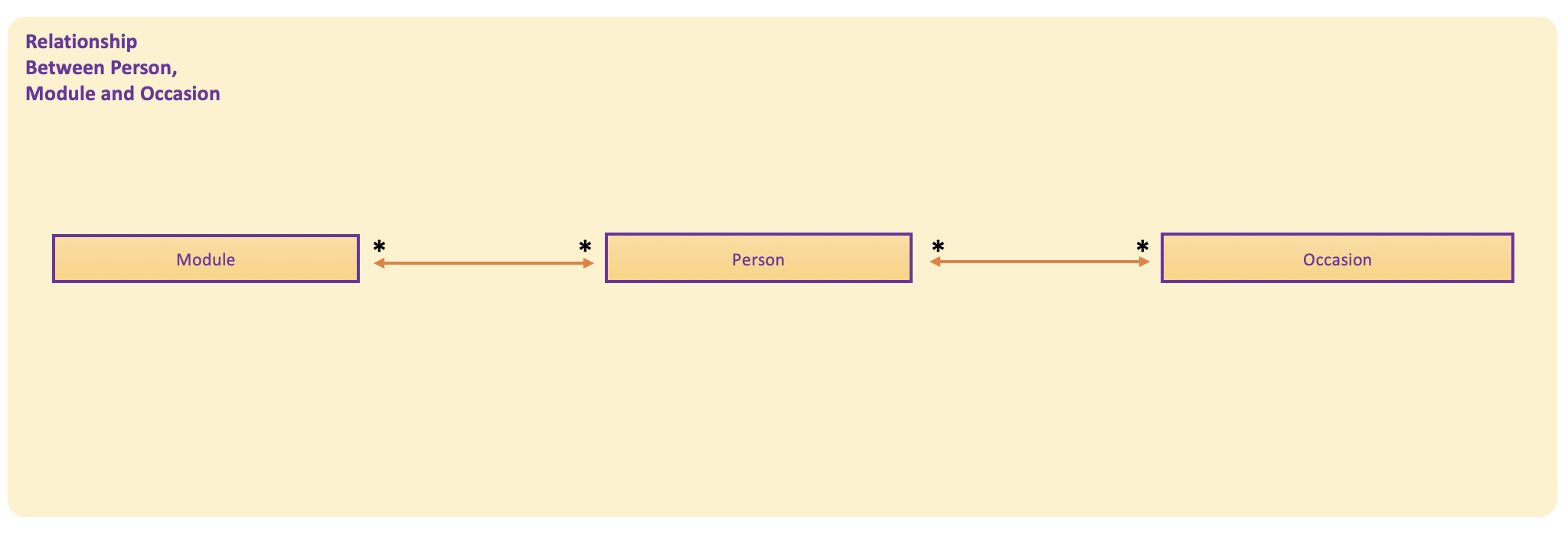
UI component
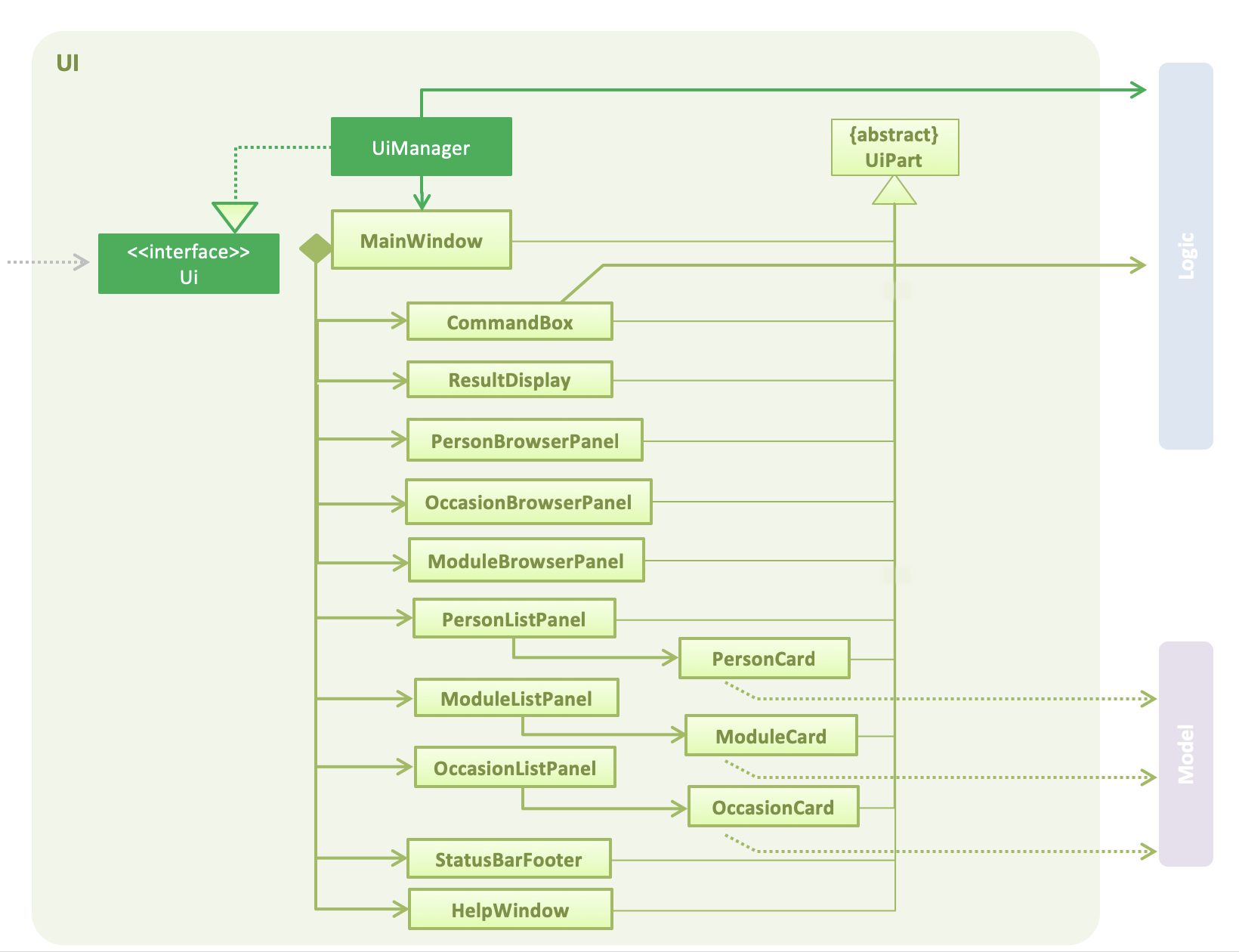
API : Ui.java
The MainWindow
is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
, BrowserPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
Duplication of Entities
To facilitate the ease of implementation of the following features, two important methods were conceived off: makeDeepDuplicate
and makeShallowDuplicate
. These two helper methods allow a Person
, Module
and Occasion
within TheTracker
to be copied in
a different manner. The following image is a depiction of how makeDeepDuplicate
works when it is called on a Person
:
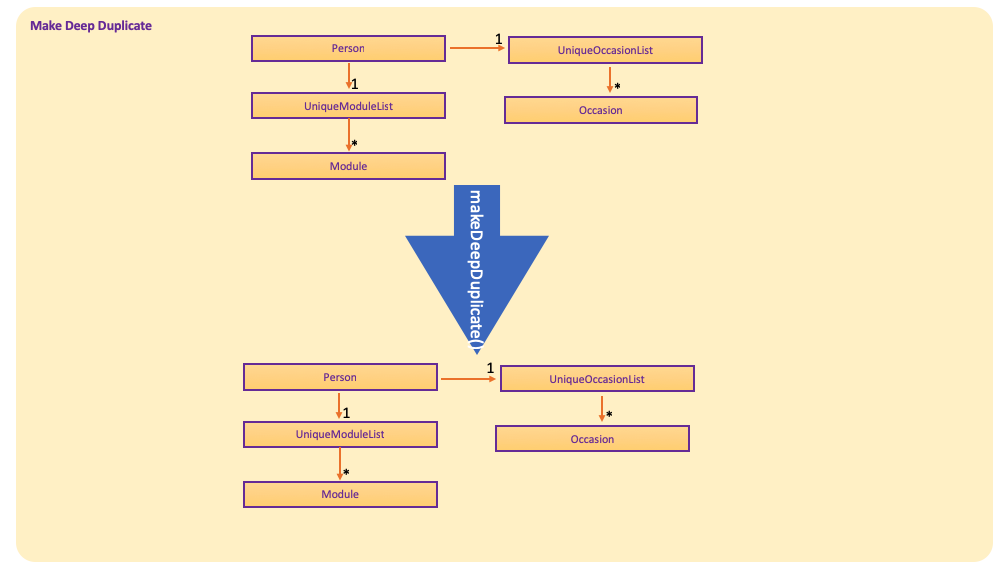
As can be seen from the above image makeDeepDuplicate
creates a replica of a Person
such that the respective Modules
within the UniqueModuleList
and
the Occasions
within the UniqueOccasionList
are also DeepDuplicated
.
The following image depicts how makeShallowDuplicate
works when it is called on a Person
within TheTracker
:
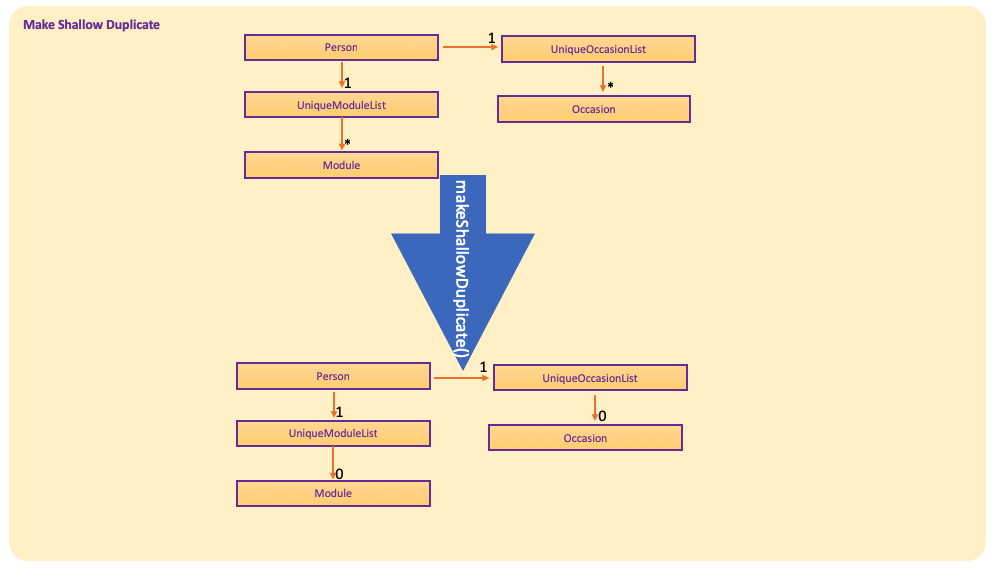
As can be seen from the above image makeShallowDuplicate
creates a replica of a Person
such that the respective UniqueModuleList
and UniqueOccasionList
are empty.
InsertPerson feature
The InsertPerson
command is an advanced feature for TheTracker. It enables a bi-directional insert of a Person into
either one of a module
or an occasion
.
Current Implementation
For the following example we will use InsertPerson
command to insert a person
, bi-directionally, into a module
.
The usage for a bi-directional insert into an occasion
is similar.
InsertPerson
command extends the Command
class and uses inheritance to facilitate implementation. It’s mechanism is
facilitated by VersionAddressBook
. Below is the associated sequence diagram for the InsertPerson
command:
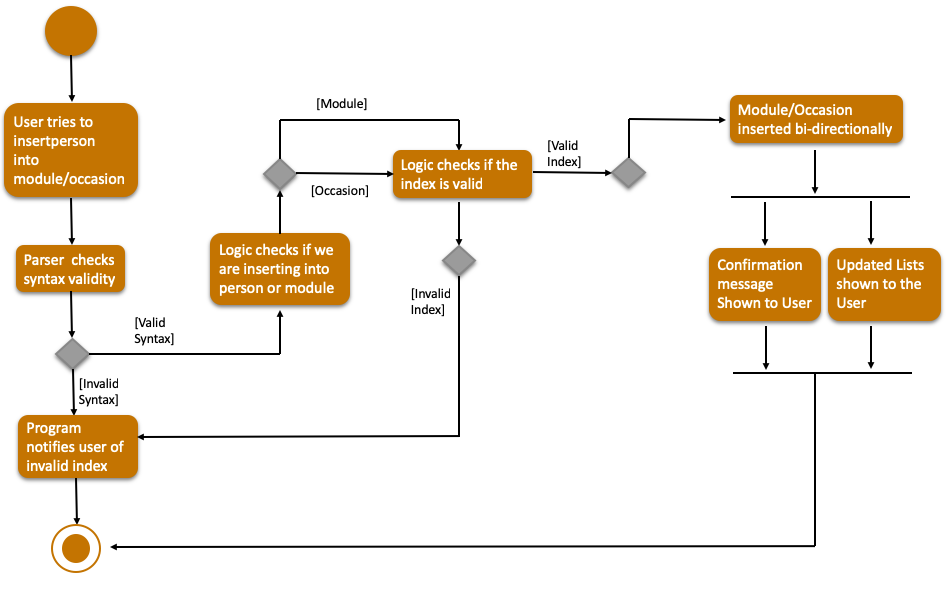
When the user first opens up TheTracker
it loads up an instance of a VersionAddressBook
and initializes
the currentStatePointer
to point at this instance of the TheTracker
. When the user then executes the InsertPerson
command an entirely new copy of the state of TheTracker
is created and inserted as a new VersionAddressBook
with the
currentStatePointer being updated. Below is a diagram that depicts this:
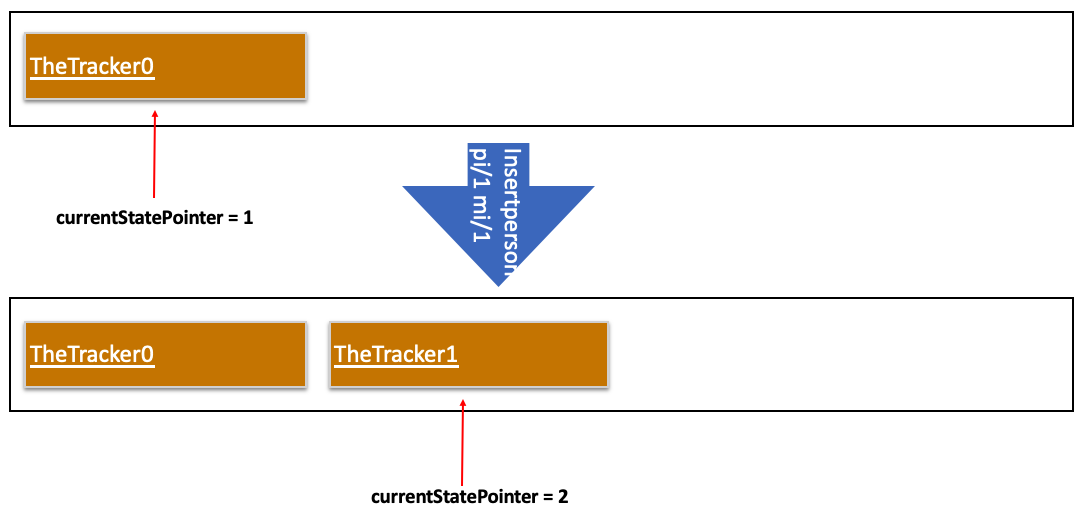
The following steps represent the internal implementation of the InsertPerson
command when inserting a Person
into a Module
:
-
Create a
DeepDuplicate
and aShallowDuplicate
of the Both thePerson
and theModule
-
Insert the
ShallowDuplicate
of the module into theDeepDuplicate
of thePerson’s
UniqueModuleList
-
Insert the
ShallowDuplicate
of the person into theDeepDuplicate
of theModule’s
UniquePersonsList
Note: Further elaboration of the above implementation is discussed within the Design Considerations
section.
Design Considerations
Why it is non-trivial:
-
For the feature to work coherently with
undo
/redo
as well asedit
an entire new replica of state ofTheTracker
must be created with the relevant entities bi-directionally inserted after this command has been executed.
We cannot, however, make do with only makeDeepDuplicate
in order to implement the InsertPerson
feature.
The following scenario illustrates why:
-
Insertion of
Person
x
intoOccasion
y
would causex’s
occasion list to be populated byy
andy’s
person list to be populated byx
-
Insertion of
Person
x
intoModule
z
would causex’s
module list to be populated byz
andz’s
person list to be populated byx
. But if we insert a deep copy ofx
intoz
this would entail us replicatingy
, which would entail us replicating the person list ofy
and, thus,x
and so the program will halt in an infinite regress until aStackOverFlow
is thrown.
To avoid the above error, upon insertion of a Module
into a Person
the respective Module’s
ShallowDuplicate
is inserted.