Overview
This document is written for summarizing my contributions in the project TheTracker.
The Tracker (v1.4) is a desktop application that helps NUS students to monitor contacts, modules and occasions. The Tracker is optimized for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI). It is designed for programmers who are used to CLI applications and are good at typing.
Summary of contributions
-
Major enhancement: Create edit features for
Module
andOccasion
and do enhancement to the existing edit person command-
What it does: allows the user to edit a person, module or occasion by index
-
Justification: This is one of the basic features of TheTracker, which allows a user to edit module and occasion in a similar way of how he edits a contact.
-
Highlights: This feature is necessary for a user to simultaneously maintain all three types of entries.
-
Credits:
-
This enhancement requires the different lists to be handled independently, but with all actions tracked simultaneously (e.g. for undo/redo).
-
This enhancement is designed to fit the insert command (All the attendence list related to the edited entity will be updated simultaneously).
-
-
-
Minor enhancement:
-
Create the export function
-
What it does: allows the user to export user data to a specified file type at a specified location.
-
Highlights: This feature enhance user’s experience to TheTracker. He can now raise a data communication (with import feature in coming v2.0) with the external world.
-
Credits: This enhancement requires the command to access storage access directly, and has restrictions on the file path user typed in.
-
-
Implement the
Occasion
class and its related classes(OccasionName
,OccasionDate
,OccasionLocation
)
-
-
Code Contributions : Code
-
Other contributions:
-
Project management:
-
Managed milestones for
v1.2
-v1.4
on Github-
Opened issues
-
Set up milestone deadlines, close issues accordingly on GitHub
-
-
-
Enhancements to existing features:
-
Community:
-
Documentation:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Editing an entry : edit
+ person
A command to allow the user to edit an existing person, module or occasion in TheTracker.
It can be used to add optional field that is not specified in the add command.
Editing a person : editperson
A command to allow the user to edit an existing person in TheTracker.
Format:
editperson INDEX [n/NAME] [p/PHONE] [e/EMAIL] [a/ADDRESS] [t/TAG]…
Example:
editperson 6 p/91234567 e/johndoe@example.com
Edits the person at INDEX 6 in TheTracker: edits his phone number to 91234567 and email address to johndoe@example.com.
Notice
-
You should edit at least one field
-
The INDEX must be valid:
-
Be a positive number
-
Not larger than the size of the person list
-
Editing a module : editmodule
A command to allow the user to edit an existing module in TheTracker.
Format:
editmodule INDEX [mc/MODULE CODE] [mt/MODULE TITLE] [ay/ACADEMIC YEAR] [sem/SEMESTER] [t/TAG]…
Example:
editmodule 1 mc/CS1101S
Edits the module of INDEX 1 in TheTracker: edits the module code to CS1101S.
Notice
-
You should edit at least one field
-
The INDEX must be valid:
-
Be a positive number
-
Not larger than the size of the module list
-
Editing an occasion : editoccasion
A command to allow the user to edit an existing occasion in TheTracker.
Format:
editoccasion INDEX [on/OCCASION NAME] [od/OCCASION DATE] [loc/OCCASION LOCATION] [t/TAG]…
Example:
editoccasion 7 on/Barbecue od/2019-6-17 loc/NUS
Edits the occasion of INDEX 7 in TheTracker: edits the occasionName to Barbecue, edits the occasionDate to 2019-6-17,
edits the occasionLocation to NUS.
Notice
-
You should edit at least one field
-
The INDEX must be valid:
-
Be a positive number
-
Not larger than the size of the occasion list
-
Exporting user data to xml/txt: export
A command to allow the user to export data from TheTracker to a specified location.
Exporting data to xml file
Export data to a xml file.
Format:
export --xml [FILEPATH]
Examples:
-
For MacOS and Linux users:
export --xml /Users/Anna/Desktop/exported.xml
-
For Windows users:
export --xml C:\Users\Anna\desktop\exported.xml
Exporting data to txt file
Export data to a txt file.
Format:
export --txt [FILEPATH]
Examples:
-
For MacOS and Linux users:
export --txt /Users/Anna/Desktop/exported.txt
-
For Windows users:
export --txt C:\Users\Anna\desktop\exported.txt
Importing data into TheTracker: import
(coming in v 2.0)
A command to allow the user to import information from external sources.
Importing xml file
Format:
import --xml [FILEPATH]
Examples:
-
For MacOS and Linux users:
import --xml /Users/Anna/Desktop/imported.xml
-
For Windows users:
import --xml C:\Users\Anna\desktop\imported.xml
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Below are the class diagrams or Person, Module and Occasion. For simplicity consideration, we divide the class diagrams in three separate ones.
Class Diagram for Person:
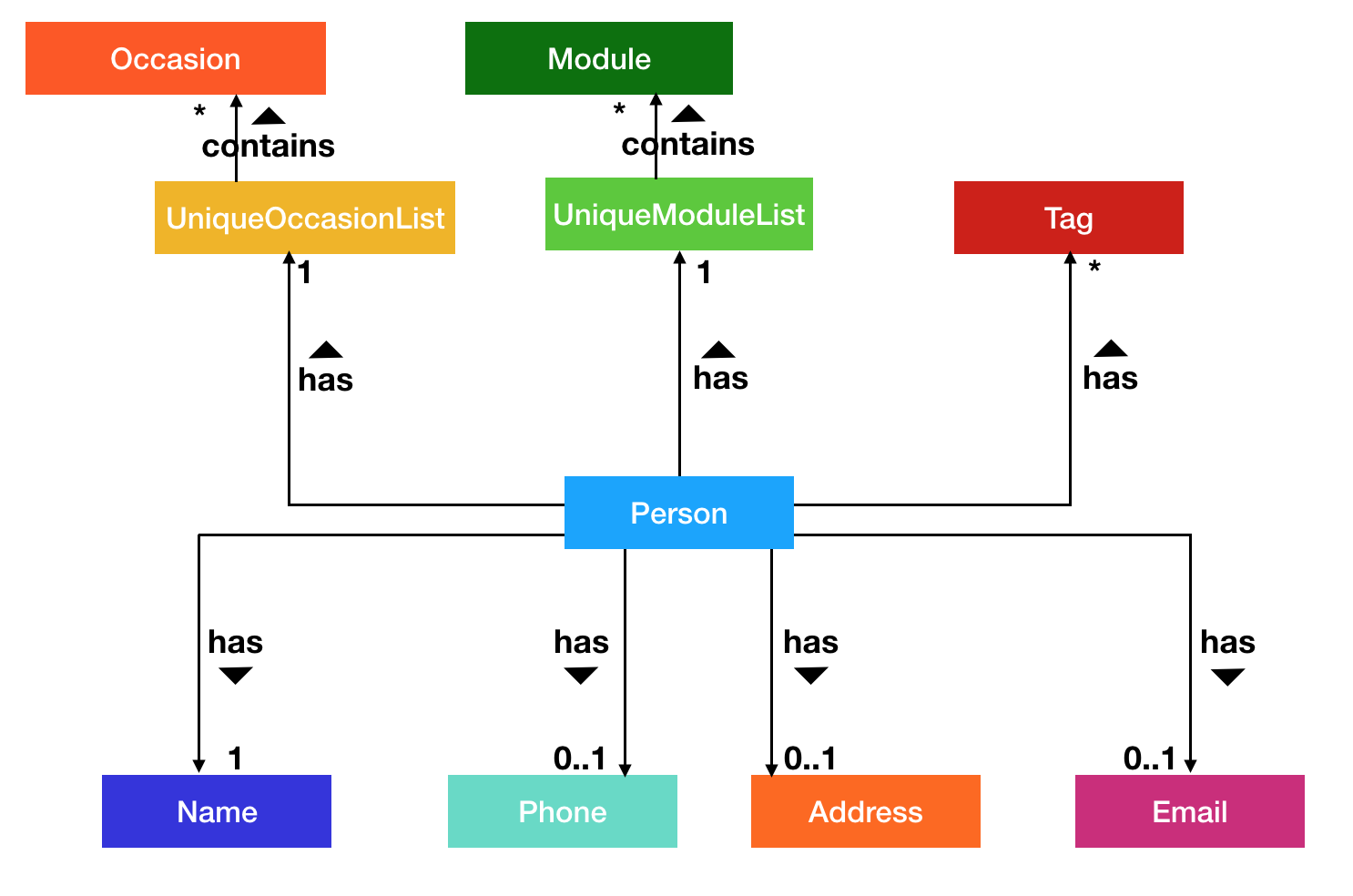
Edit Person/Module/Occasion Feature
edit
is a basic feature for TheTracker
.
It supports editing of Person
, Module
and Occasion
by command editperson
,
editmodule
and editoccasion
respectively.
Current Implementation
The following section will use EditPersonCommand
as an example to explain the implementation.
EditModuleCommand
and EditOccasionCommand
use the same mechanism as EditPersonCommand
.
EditPersonCommand
extends the Command
class and uses inheritance to facilitate implementation.
It’s mechanism is facilitated by VersionAddressBook
. In addition, it implements the following operations:
-
VersionedAddressBook#commit()
: Saves the current book state in the command history -
VersionedAddressBook#updatePerson(target, editedPerson)
: Update the targetedPerson with edited fields -
ModelManager#updateFilteredPersonList(Predicate<Person> predicate)
: Returns an unmodifiable view of the list of Person backed by the internal list of VersionedAddressBook
These operations are exposed in the Model interface as
Model#commitAddressBook()
, Model#updatePerson(target, editedPerson)
and Model#updateFilteredPersonList(Predicate<Person> predicate)
respectively.
The following sequence diagram shows how the editperson
operation works:
editperson 6 p/91234567 e/johndoe@example.com
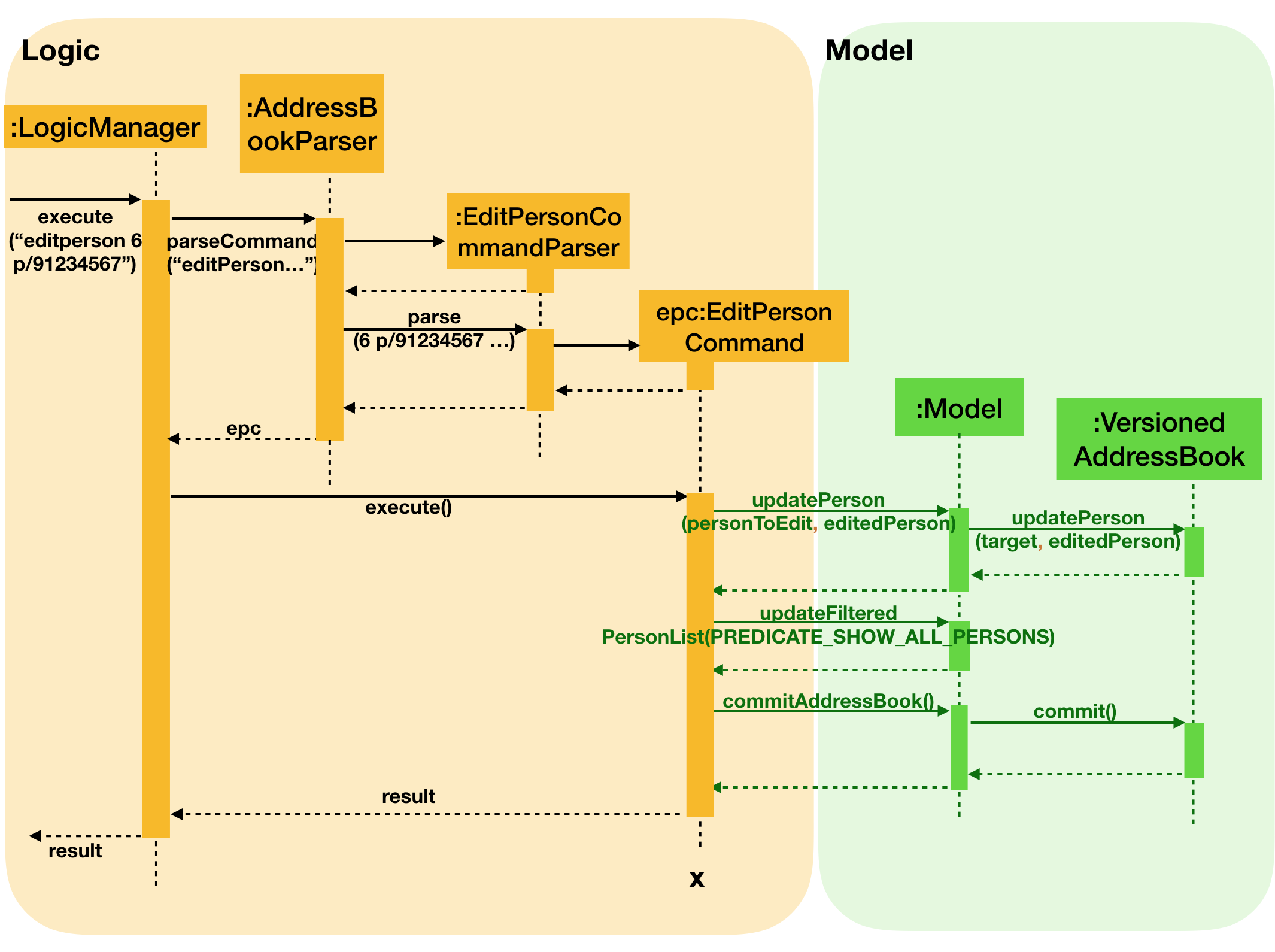
editmodule
and editoccasion
works in a similar way as editperson
The mechanism for modifying edit
commands to fit insert
commands is similiar to and explained in delete
command
Design Considerations
Aspect: How editperson/editmodule/editoccasion executes
-
Alternative 1 (Current Implementation):
EditPersonCommand
,EditModuleCommand
andEditOccasionCommand
are three separate classes-
Pros:
-
Implementation is easy to understand
-
Easy to manage the three entity lists
-
-
Cons:
-
Lack of the essence of polymorphism, which is a key feature in OOP
-
-
-
Alternative 2 : Let
EditPersonCommand
,EditModuleCommand
andEditOccasionCommand
inherits fromEditCommand
, then useTypeUtil
to detect the kind of entity that is going to be edited-
Pros:
-
A good utilisation of polymorphism
-
-
Cons:
-
Person
,Module
,Occasion
are three relatively independent entities, it could be hard to handle when using polymorphism and put everything together
-
-
Export
Export is a feature to enhance the functionality of TheTracker. TheTracker (v1.4) supports exporting user data to xml file and txt file.
Current Implementation
ExportXmlCommand
and ExportTxtCommand
are both inherited from ExportCommand
, which inherits from Command
class
In order to gain a direct access to the Storage
component, the method setStorage(Storage storage)
is added to Command
In order to reduce redundant code in commands that are not directly dependent on Storage
, setStorage(Storage storage)
is
designed to be a non-abstract method. Only ExportCommand
overrides this method.
For ExportTxtCommand
ExportTxtCommand
can be regarded as an extension of ExportXmlCommand
.
This interaction of ExportTxtCommand
and Storage
component works in the same way of ExportXmlCommand
. The difference is: ExportTxtCommand
first stored the exported xml file temp.xml
;
then, the method XmlToTxtUtil.parse()
will parse the xml file to a txt file with defaulted style and layout
and stored in the exportedFilePath
specified by the user.
Design Considerations
Aspect: The role of exported file type
-
As a parameter (current implementation):
-
Format:
export --xml [FILEPATH]
andexport --txt [FILEPATH]
-
Pros:
-
Can utilise polymorphism
-
Can avoid unnecessary repetition of code
-
-
Cons:
-
Not consistent with the format of other commands (command + parameterPrefix + parameter)
-
-
-
As a part of command :
-
Format:
exportxml fp/[FILEPATH]
andexporttxt fp/[FILEPATH]
-
Pros:
-
Can keep consistent with the format of other command (command + parameterPrefix + parameter)
-
-
Cons:
-
ExportXmlCommand
andExportTxtCommand
have to be two independent commands, which does not utilise polymorphism -
Have unnecessary repetition of code
-
-
Aspect: The Location of valid filepath check
-
Check in
ExportCommand
(current implementation) :-
Pros:
-
As only export commands are directly related to external file path that the user types in, which needs to check validation, checking in
ExportCommand
and its subclasses can avoid unnecessary checking. -
Easy to implement and clear to understand
-
-
Cons:
-
Can only check the validation of external file path that the user types in
-
-
-
Check in
AddressBookStorage
:-
Pros:
-
Can check the validation of all the file paths occurred in
Storage
component of this project
-
-
Cons:
-
Have unnecessary checking of the validation of file paths
-
-